Java Intro #
This lab will walk you through setting up the IntelliJ Integrated Development Environment (IDE) and introduce you to the the basics of Java.
[0] Setup #
GitHub Configuration #
๐ป Join the isf-dp-cs organization on GitHubYou will have received an email inviting you to join. You can also log in to github.com to accept the invitation.
๐ป Select
[Projects
] > [Get from VCS
]
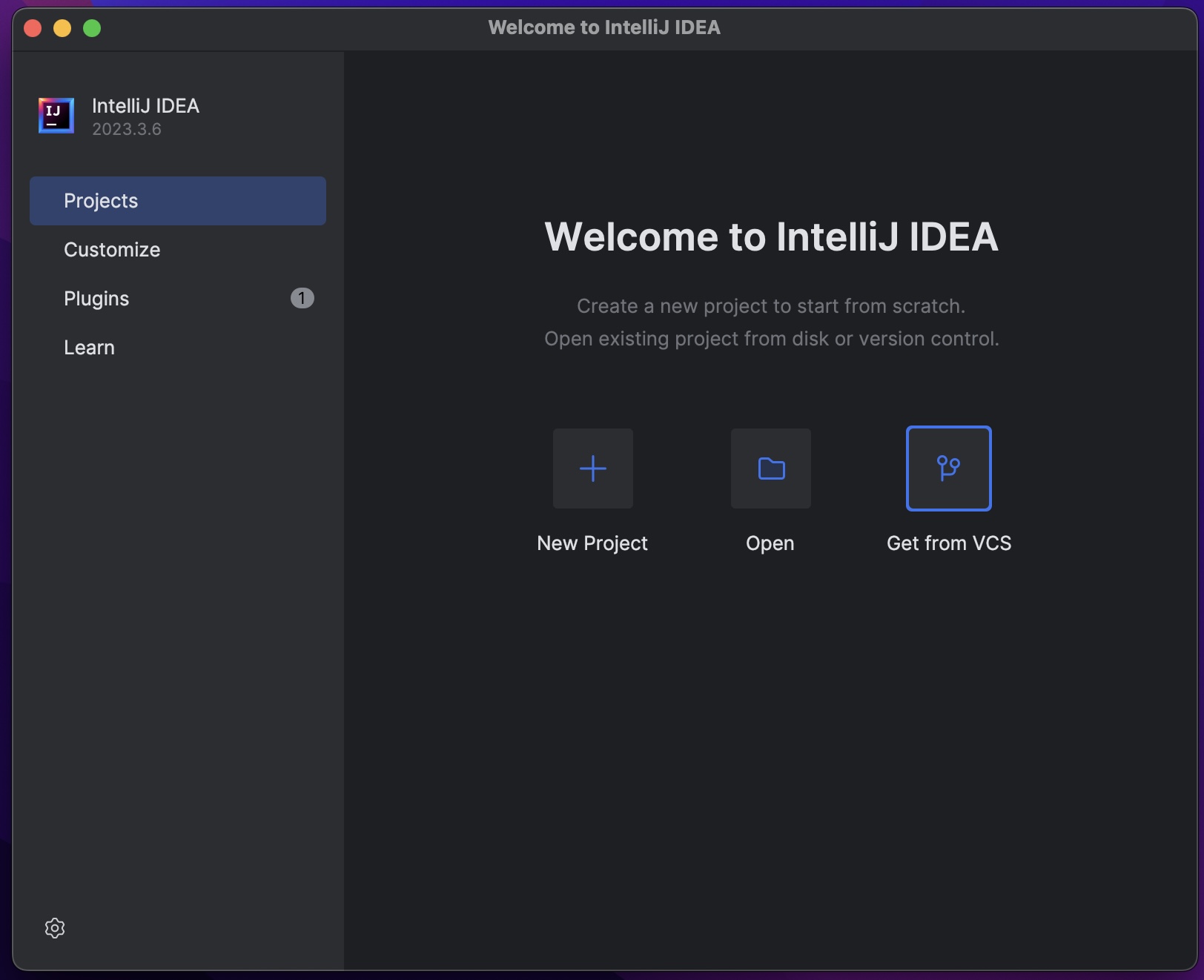
๐ป Select
[Github
]
๐ป Click
[Authorize
]
Clone the Repository #
๐ป select [Projects
] > [Get from VCS
] > [Repository URL
]
#
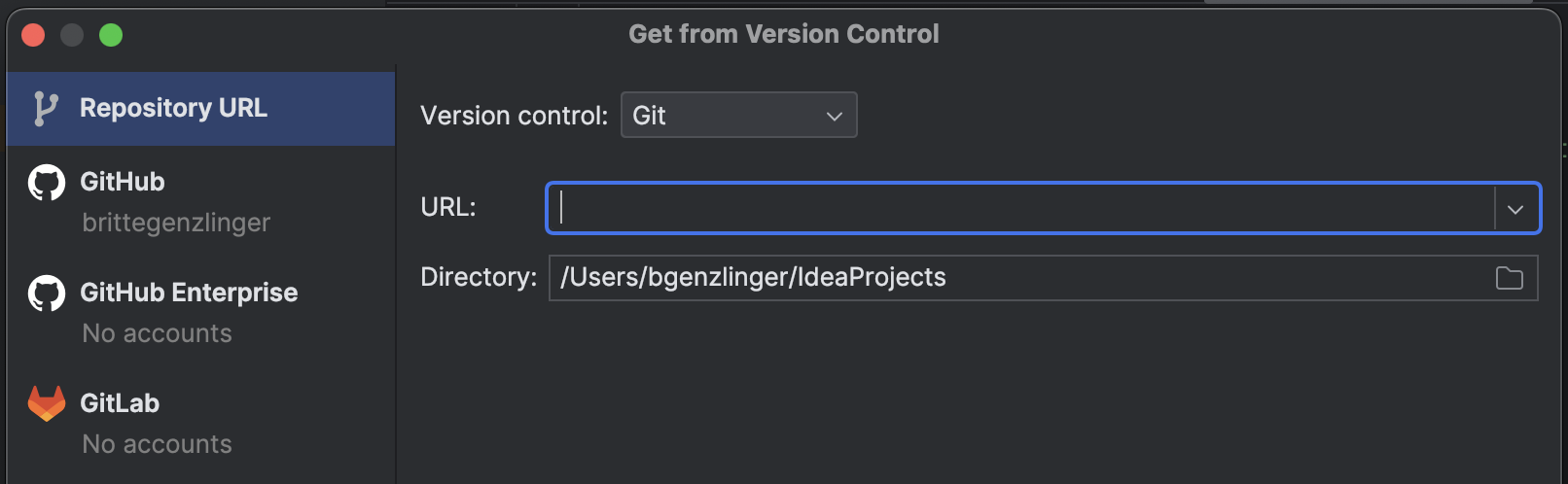
Be sure to change yourgithubusername
to your actual GitHub username.
https://github.com/isf-dp-cs/lab_intro_yourgithubusername
[1] Variables #
In Java, you have to specify the data type of each variable as you declare it, like this:
int videosWatched = 353;
Java has 8 primitive data types:
Data Type | Size | Description |
---|---|---|
byte | 1 byte | Stores whole numbers from -128 to 127 |
short | 2 bytes | Stores whole numbers from -32,768 to 32,767 |
int | 4 bytes | Stores whole numbers from -2,147,483,648 to 2,147,483,647 |
long | 8 bytes | Stores whole numbers from -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
float | 4 bytes | Stores fractional numbers. Sufficient for storing 6 to 7 decimal digits |
double | 8 bytes | Stores fractional numbers. Sufficient for storing 15 decimal digits |
boolean | 1 bit | Stores true or false values |
char | 2 bytes | Stores a single character/letter or ASCII values |
You can print variables and text to the console like this:
System.out.println("Number of videos watched: " + videosWatched);
๐ป Add each of the following variables to your code, and print each one to the console. Be sure to use an appropriate data type.
// add a variable that stores your username
// add a variable that stores the number of videos youve uploaded
// add a variable that stores the average rating you give to videos
// add a variable that stores the theme setting as 'D' 'L' or 'A' for dark mode, light mode, or auto
// add a variable that stores whether you are signed in or not
// add a variable that stores the name of your country
[2] Strings #
Strings
are more complex objects that come with certain features. Here are some examples:
String firstName = "John";
String lastName = "Doe";
System.out.println(firstName + " " + lastName); // add Strings together
String txt = "Hello! World";
int length = txt.length(); // get the length of a String
String first = "java programming";
String second = "java programming";
boolean result1 = first.equals(second); // compare first and second Strings
String txt = "Hello World";
char letter = txt.charAt(2); // returns character at index 2
ANSI Codes #
ANSI escape codes are special codes that can change the formatting when you print to the console
String yellowBackground = "\u001b[43;1m";
String reset = "\u001b[0m";
System.out.println(yellowBackground + "the sun is yellow" + reset);
The 256 Background Colors follow a simple forumula: \u001b[48;5;
+ n + m
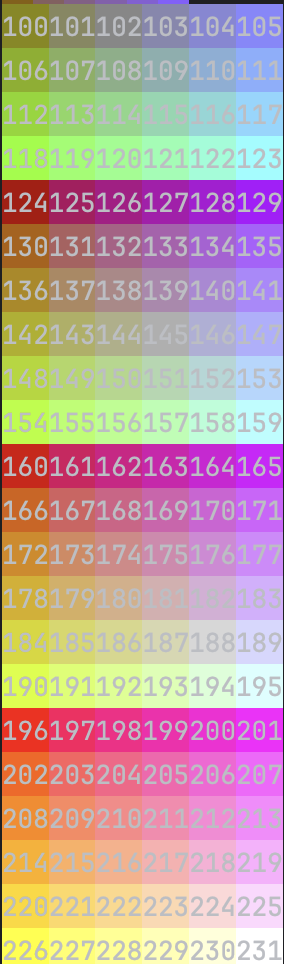
[3] Conditionals #
User Input #
In java, you get user input like this:
Scanner scanner = new Scanner(System.in); // create a Scanner object
System.out.print("Are you having a good class so far? (y/n)");
String input = scanner.nextLine(); // get the user input
Modulo #
Just like in pseudocode, we can use the modulo operator to calculate the remainder of division. In java, we will use the %
operator for mod.
int a = 15;
int b = 8;
int remainder = a % b; // calculate the remainder
๐ป Create a new loop in the code that it will do the following:
- ask the user for their name
- ask the user for their favorite color
- ask the user for their second favorite color
- print out the user’s name, alternating between the two colors for each letter
[4] Wordle #
Now it’s time to put all your new skills to good use! You will be coding the game Wordle
.
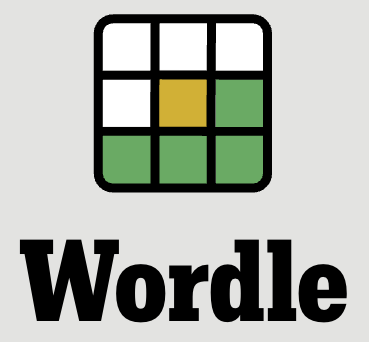
Looping #
Right now, the code picks a random 5-letter word, and allows the user a single guess. Not much of a game!
๐ป Add a loop to the code, so that the user gets 6 guesses. You can reference your code from the other files to write your loop.
Highlight #
A big part of Wordle
is the feedback from the game. After each guess, the user is shown their guess, and each letter is highlighted according to these rules:
- GRAY backround: guess letters not included in the word
- YELLOW backround: guess letters in the wrong location
- GREEN backround: guess letters in the correct location
End the loop early #
Right now, the user will be asked for 6 guesses no matter what. However, if they guess correctly, the loop should end early. Here are three examples of while
loops:
// java while loop
while (i < 6) {
System.out.println("Hello World");
i++;
}
// java while loop with OR logic
while (i < 6 || i < 1) {
System.out.println("Hello World");
i++;
}
// java while loop with AND logic
while (i < 6 && i > 0) {
System.out.println("Hello World");
i++;
}
[5] Deliverables #
โกโจ๐ป Push your code to GitHub.
Include a descriptive commit message.
โ If you would like teacher feedback, begin your commit message with
#feedback